-
Notifications
You must be signed in to change notification settings - Fork 186
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Memory Leak + Reduced Framerate in 0.7 #218
Comments
I can't say I suggest hiding and showing a grid by spawing and despawing the grid but I still think this is a valid issue. We do a lot of caching on the rendering side and I have a feeling something isn't being cleaned up correctly. |
@StarArawn Thanks for the response. I agree with the suggestion about despawn/respawn on toggle. I think the reason why this issue concerned me is I am currently working on an "infinite" 2d map using noise, and it would use a chunking design somewhat similar to the I was able to reproduce this same issue with the Thanks again for the response, I'm not super familiar with the custom render pipeline, but I have some time in the morning that I can poke around. Would love to contribute anyway I can. |
I've looked into this more closely and this is not an issue with |
One solution as a workaround is to avoid despawning entities and attempt to reuse them instead. For example if you mark a chunk as visible false and then when a new chunk spawns you can pull that chunk in(via a marker component?) change its tiles and transform to the new location. Hopefully this issue is fixed soon in bevy with the release of 0.8.1 :) |
The memory leak might indeed be on my side I'm looking for it now. |
Profiling heap usage isn't exactly straight-forward on windows, so I'm still working on that, but just based on some debug logs I added in prepare and extract, I wasn't able to locate anything that seemed like a leak. Were you able to find anything? |
There were two hash maps for mapping entities to tile positions but I clear that data now and I still get like 10mb's of leak in a modified chunking example. Still trying to narrow down where. |
I was able to figure out the issue. Although some memory does still leak somewhere.. In order to use I've opened up a PR with some fixes here: |
Not sure whether or not this helps, but I was able to get a dhat allocator running against the chunking example: You can open the The entries with
|
Fixed by #219! |
Might I also add that the "small remaining leak" that seemed to still be present is present in a blank bevy application as long as you leave it running long enough, you'll see memory slowly increase. However, this appears to only exist in debug mode. |
This issue appears to be related to the Tilemap plugin, but I did upgrade to bevy 0.8 and this plugin simultaneously, and it's somewhat unclear where this issue might exist.
The code in question uses a
G
keypress to toggle a "debug grid" tilemap entity over the world tile map.The
map_size
in this case is: 160x160 with atile_size
of 50x50. The tile images are under a non-sharable license, but I can crop the debug tiles if necessary (just let me know).Here's a video from one of my streams where I can demonstrate the problem occurring. I originally noticed the framerate dropping significantly when constantly toggling, and then discovered that memory tends to increase each time the grid is toggled as well.
bevy-0.8-tilemap-leak.mp4
I also generated a Tracy profile, which just shows that everything in bevy tends to slow down each time the grid is toggled. Here are the trace times towards the end of my test (the last 10s):
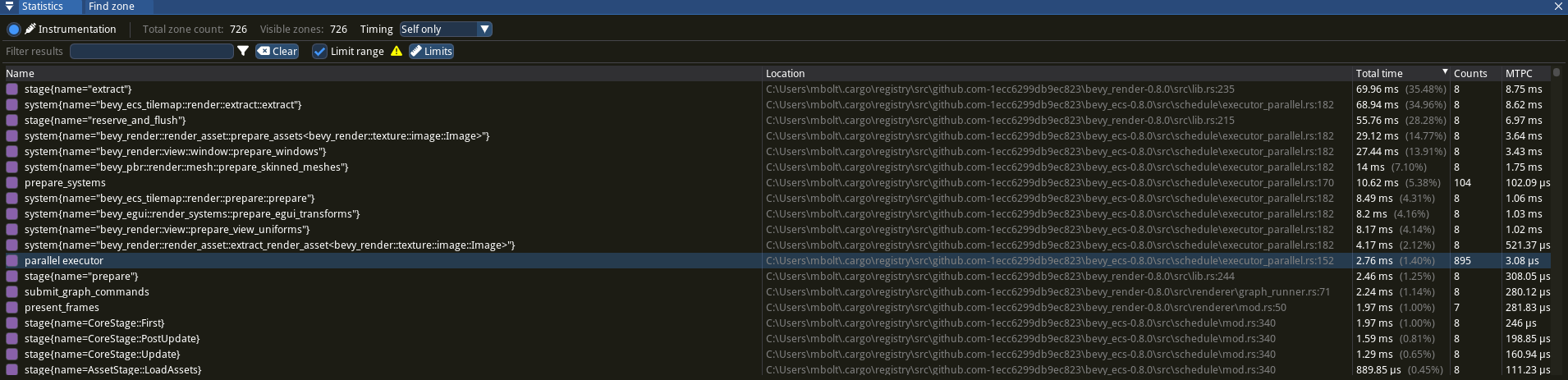
Here are the frame times associated with the full run:

I hosted the tracy-profile on google drive (it's 114mb) if you're interested: https://drive.google.com/file/d/1uHzEi_eZWyEYL-GNaQ415cLHIdX58iZM/view?usp=sharing
Thanks again for a wonderful project. I've had an awesome experienced building my game using this plugin. The new API is highly intuitive, loving it so far.
The text was updated successfully, but these errors were encountered: