New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
How to preserve an audio's pitch after changing AudioBufferSourceNode.playbackRate? #53
Comments
I attempted to use the In this code, we define 3 melodies and transpose it to every key for a long playing sample. These two lines within
work when used independently. But when used together, as shown, neither one takes effect. So they appear to exhibit exclusive usage.
|
I changed AudioBufferSourceNode.playbackRate using the code here https://github.com/mdn/webaudio-examples/blob/master/offline-audio-context-promise/index.html but found out that the pitch was not preserved.
I looked up the documentation and found out that there is no pitch correction when using AudioBufferSourceNode.playbackRate.
If we change an audio's playbackRate using HTML audio element, the pitch would be preserved. Is there a way that I can obtain the same effect?
https://developer.mozilla.org/en-US/docs/Web/API/AudioBufferSourceNode
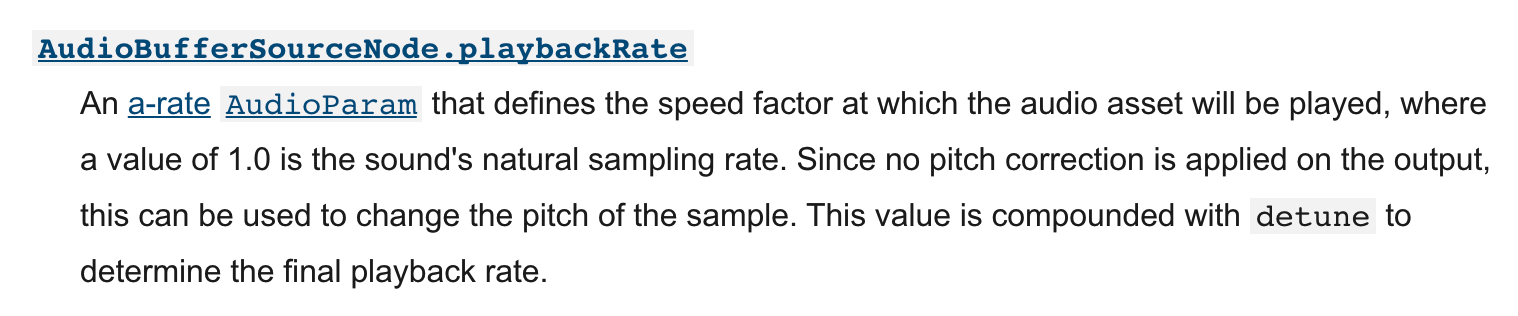
The text was updated successfully, but these errors were encountered: