New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We鈥檒l occasionally send you account related emails.
Already on GitHub? Sign in to your account
[Question] Great Library ! How to intercept Motion data #247
Comments
Your import React, { useEffect } from 'react';
import { Canvas, useFrame, useThree } from '@react-three/fiber';
import { useController } from '@react-three/xr';
const WebXr = () => {
const { gl } = useThree();
const leftController = useController('left');
const rightController = useController('right');
const gazeController = useController('gaze');
useEffect(() => {
const handleControllerInput = (event) => {
console.log(event.target.name, event.target.position.toArray(), event.target.rotation.toArray());
};
leftController.addEventListener('selectstart', handleControllerInput);
rightController.addEventListener('selectstart', handleControllerInput);
gazeController.addEventListener('selectstart', handleControllerInput);
return () => {
leftController.removeEventListener('selectstart', handleControllerInput);
rightController.removeEventListener('selectstart', handleControllerInput);
gazeController.removeEventListener('selectstart', handleControllerInput);
};
}, [leftController, rightController, gazeController]);
useFrame(() => {
gl.xr.isPresenting && console.log(leftController.position.toArray(), rightController.position.toArray(), gazeController.position.toArray());
});
return (
<mesh position={[0, 0, -3]}>
<boxBufferGeometry args={[2, 2, 2]} />
<meshBasicMaterial color="white" />
</mesh>
);
};
export default () => (
<Canvas>
<WebXr/>
</Canvas>
); |
I think I understand and appreciate what you tried to do with the default export ... Might there be a working example which does the same thing ? Maybe just for the headset ? |
I'd see our examples: Lines 1 to 61 in be18055
|
I tried a few examples and they seemed to work very well.
This one I'm a bit stumped 馃
It seems very simple - I'm just trying to intercept the position and orientation of a Quest 2 headset and left/right controllers.
It seems to transpile fine, but in the console I'm getting ...
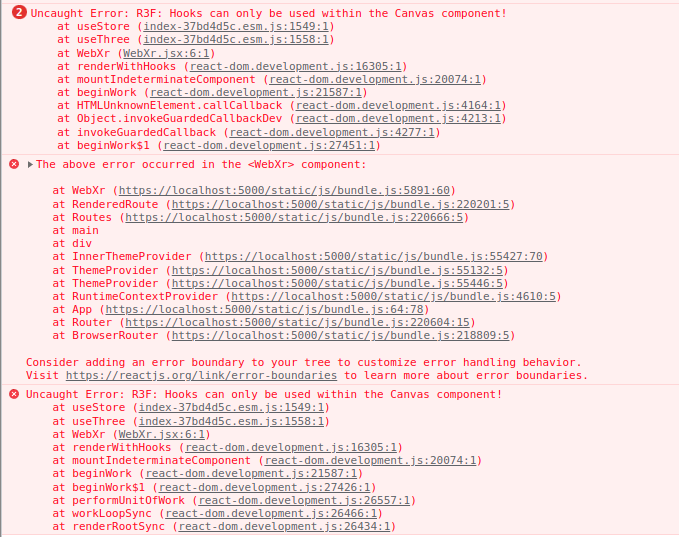
It's all being used in Canvas no?
Or, am I thinking about this wrong - do I need references in other sub components?
Is there a simple example which prints the data I'm looking for to console, it seems to have eluded me...
Any help is most appreciated :)
The text was updated successfully, but these errors were encountered: