forked from bevyengine/bevy
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
separate tonemapping and upscaling passes (bevyengine#3425)
Attempt to make features like bloom bevyengine#2876 easier to implement. **This PR:** - Moves the tonemapping from `pbr.wgsl` into a separate pass - also add a separate upscaling pass after the tonemapping which writes to the swap chain (enables resolution-independant rendering and post-processing after tonemapping) - adds a `hdr` bool to the camera which controls whether the pbr and sprite shaders render into a `Rgba16Float` texture **Open questions:** - ~should the 2d graph work the same as the 3d one?~ it is the same now - ~The current solution is a bit inflexible because while you can add a post processing pass that writes to e.g. the `hdr_texture`, you can't write to a separate `user_postprocess_texture` while reading the `hdr_texture` and tell the tone mapping pass to read from the `user_postprocess_texture` instead. If the tonemapping and upscaling render graph nodes were to take in a `TextureView` instead of the view entity this would almost work, but the bind groups for their respective input textures are already created in the `Queue` render stage in the hardcoded order.~ solved by creating bind groups in render node **New render graph:** 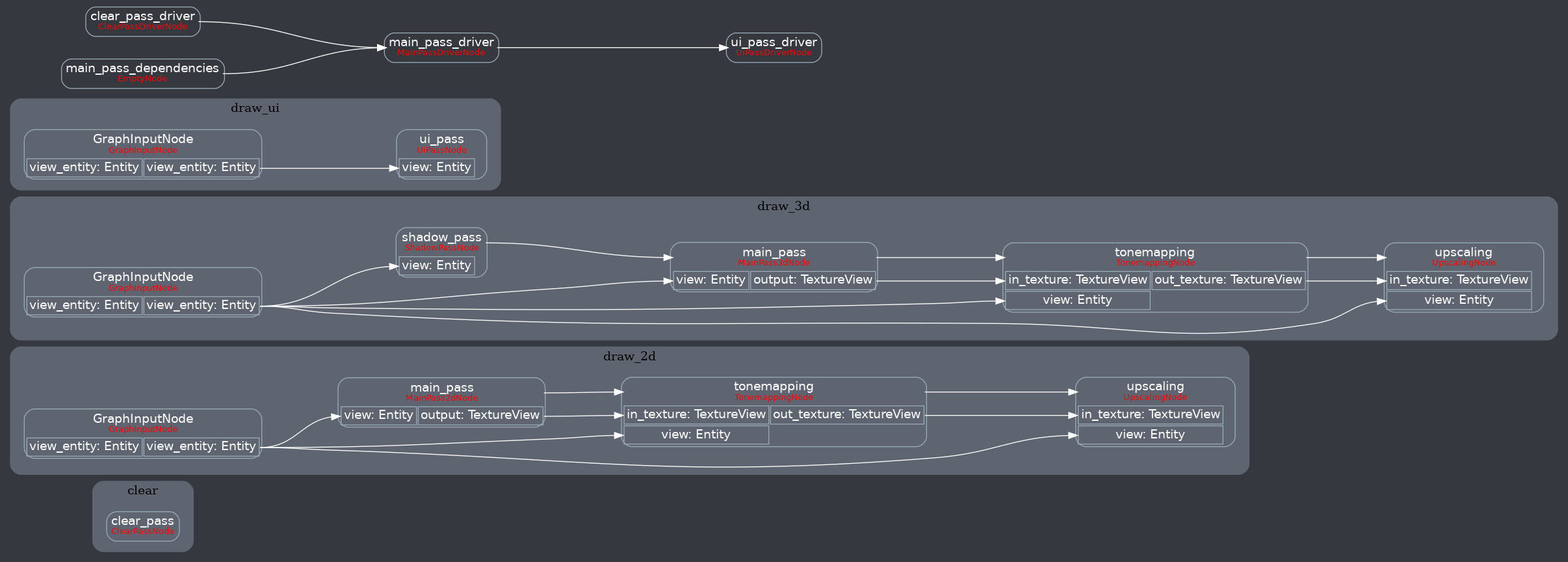 <details> <summary>Before</summary> 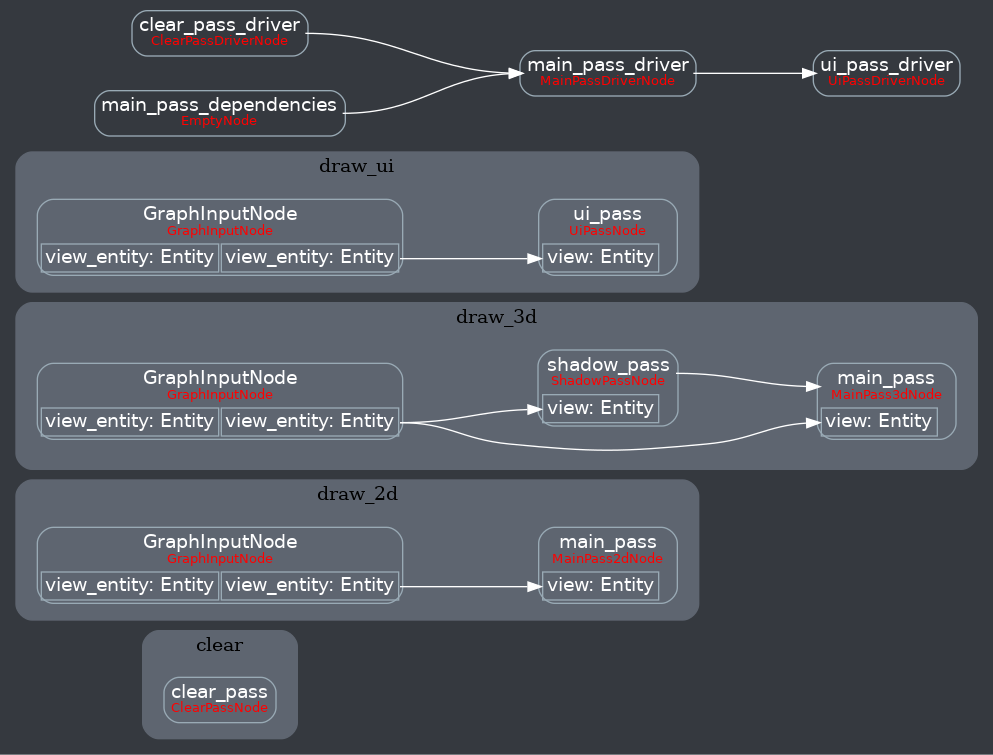 </details> Co-authored-by: Carter Anderson <mcanders1@gmail.com>
- Loading branch information
Showing
36 changed files
with
1,142 additions
and
216 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
16 changes: 16 additions & 0 deletions
16
crates/bevy_core_pipeline/src/fullscreen_vertex_shader/fullscreen.wgsl
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
#define_import_path bevy_core_pipeline::fullscreen_vertex_shader | ||
|
||
struct FullscreenVertexOutput { | ||
@builtin(position) | ||
position: vec4<f32>, | ||
@location(0) | ||
uv: vec2<f32>, | ||
}; | ||
|
||
@vertex | ||
fn fullscreen_vertex_shader(@builtin(vertex_index) vertex_index: u32) -> FullscreenVertexOutput { | ||
let uv = vec2<f32>(f32(vertex_index >> 1u), f32(vertex_index & 1u)) * 2.0; | ||
let clip_position = vec4<f32>(uv * vec2<f32>(2.0, -2.0) + vec2<f32>(-1.0, 1.0), 0.0, 1.0); | ||
|
||
return FullscreenVertexOutput(clip_position, uv); | ||
} |
26 changes: 26 additions & 0 deletions
26
crates/bevy_core_pipeline/src/fullscreen_vertex_shader/mod.rs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,26 @@ | ||
use bevy_asset::HandleUntyped; | ||
use bevy_reflect::TypeUuid; | ||
use bevy_render::{prelude::Shader, render_resource::VertexState}; | ||
|
||
pub const FULLSCREEN_SHADER_HANDLE: HandleUntyped = | ||
HandleUntyped::weak_from_u64(Shader::TYPE_UUID, 7837534426033940724); | ||
|
||
/// uses the [`FULLSCREEN_SHADER_HANDLE`] to output a | ||
/// ```wgsl | ||
/// struct FullscreenVertexOutput { | ||
/// [[builtin(position)]] | ||
/// position: vec4<f32>; | ||
/// [[location(0)]] | ||
/// uv: vec2<f32>; | ||
/// }; | ||
/// ``` | ||
/// from the vertex shader. | ||
/// The draw call should render one triangle: `render_pass.draw(0..3, 0..1);` | ||
pub fn fullscreen_shader_vertex_state() -> VertexState { | ||
VertexState { | ||
shader: FULLSCREEN_SHADER_HANDLE.typed(), | ||
shader_defs: Vec::new(), | ||
entry_point: "fullscreen_vertex_shader".into(), | ||
buffers: Vec::new(), | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
#import bevy_core_pipeline::fullscreen_vertex_shader | ||
|
||
@group(0) @binding(0) | ||
var texture: texture_2d<f32>; | ||
@group(0) @binding(1) | ||
var texture_sampler: sampler; | ||
|
||
@fragment | ||
fn fs_main(in: FullscreenVertexOutput) -> @location(0) vec4<f32> { | ||
return textureSample(texture, texture_sampler, in.uv); | ||
} |
Oops, something went wrong.