forked from console-rs/console
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat(console): display warnings in task details *and* list (console-r…
…s#123) The current method of displaying warnings is as list of all individual tasks with warnings on the task list screen. As discussed in the comments on PR console-rs#113, this may not be the ideal approach when there are a very large number of tasks with warnings. This branch changes this behavior so that the details of a particular warning for a given task is shown only on the task details screen. On the task list screen, we instead list the different _categories_ of warnings that were detected, along with the number of tasks with that warning. This means that when a large number of tasks have warnings, we will only use a number of lines equal to the number of different categories of warning that were detected, rather than the number of individual tasks that have that warning. Each individual task that has warnings shows a warning icon and count in a new column in the task list table. This makes it easy for the user to find the tasks that have warnings and get details on them, including sorting by the number of warnings. Implementing this required some refactoring of how warnings are implemented. This includes: * Changing the `Warn` trait to have separate methods for detecting a warning, formatting the warning for an individual task instance, and summarizing the warning for the list of detected warning types * Introducing a new `Linter` struct that wraps a `dyn Warning` in an `Rc` and clones it into tasks that have lints. This allows the task details screen to determine how to format the lint when it is needed. It also allows tracking the total number of tasks that have a given warning, by using the `Rc`'s reference count. ## Screenshots To demonstrate how this design saves screen real estate when there are many tasks with warnings, I modified the `burn` example to spawn several burn tasks rather than just one. Before, we spent several lines on warnings (one for each task): 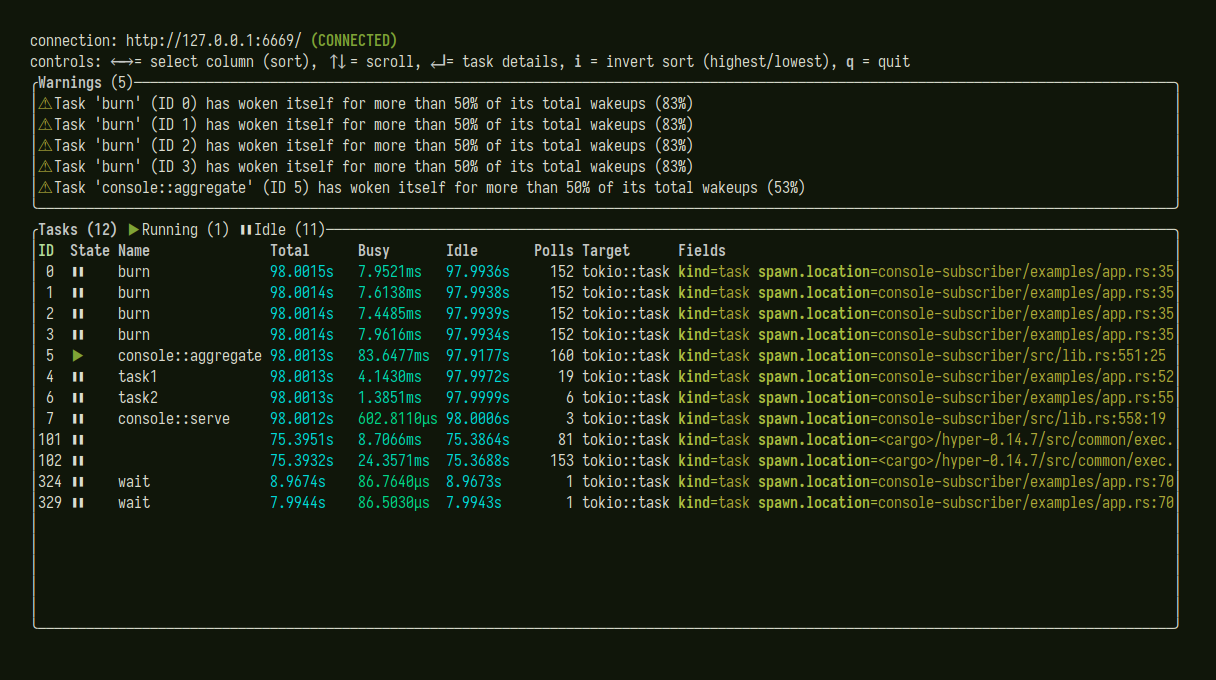 After, we only need one line: 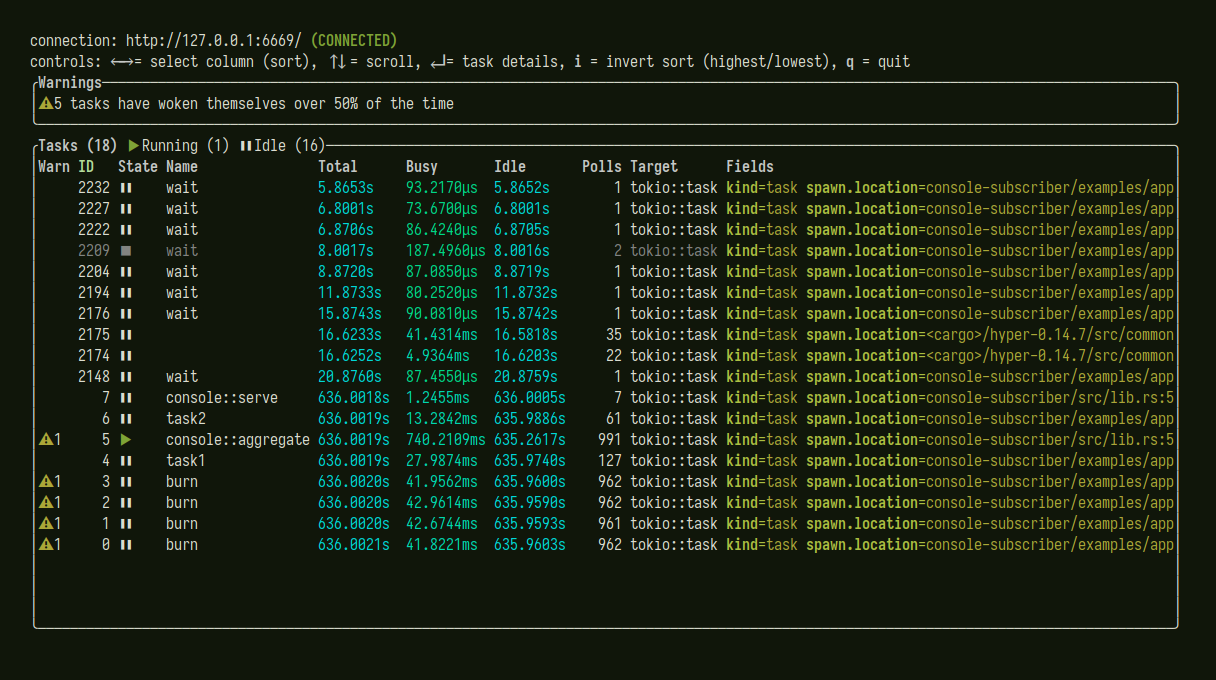 The detailed warning text for the individual tasks are displayed on the task details view: 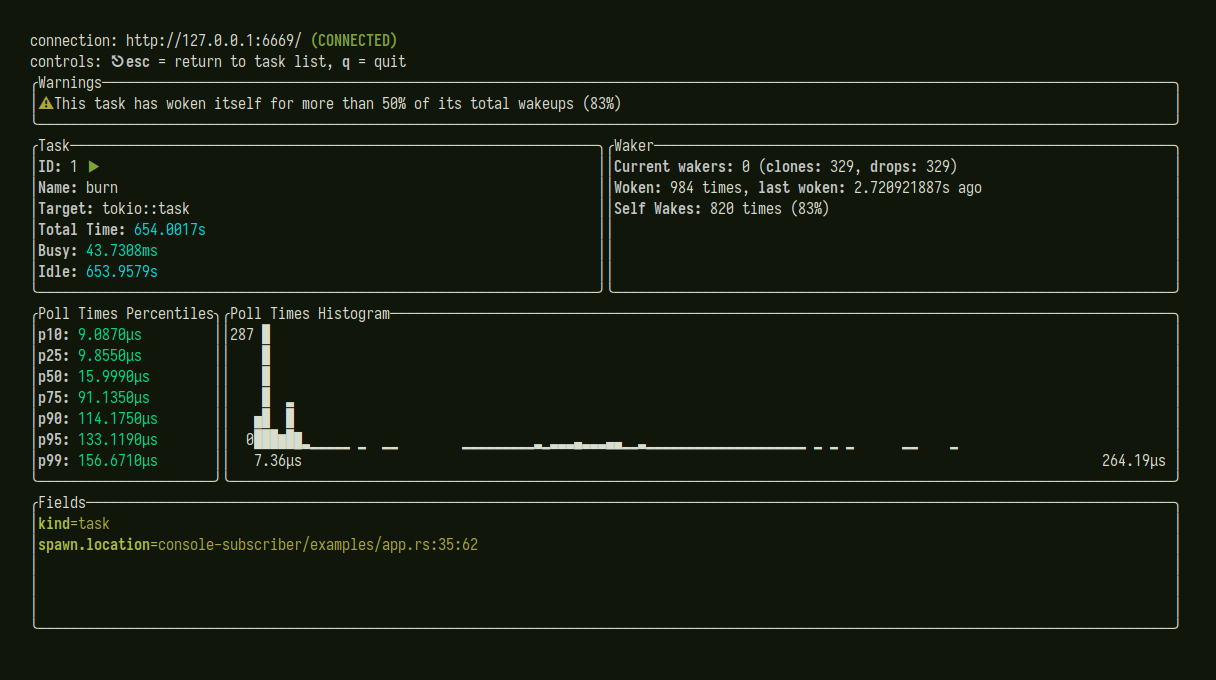 And, it still looks okay in ASCII-only mode: 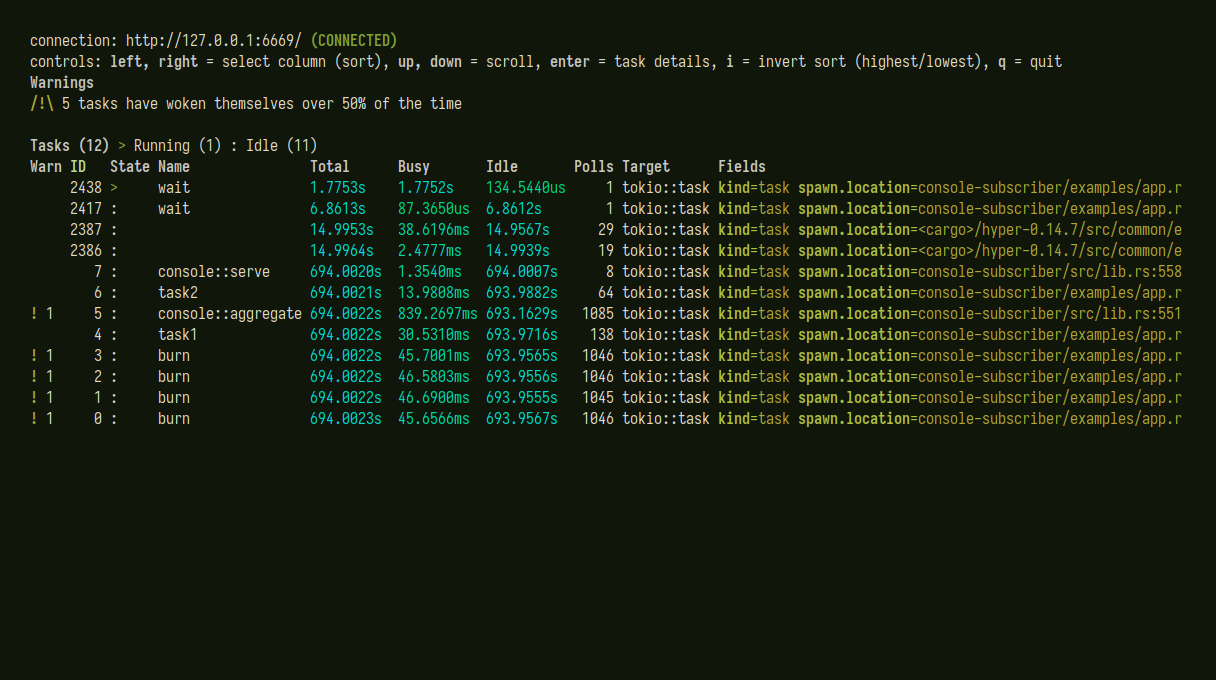 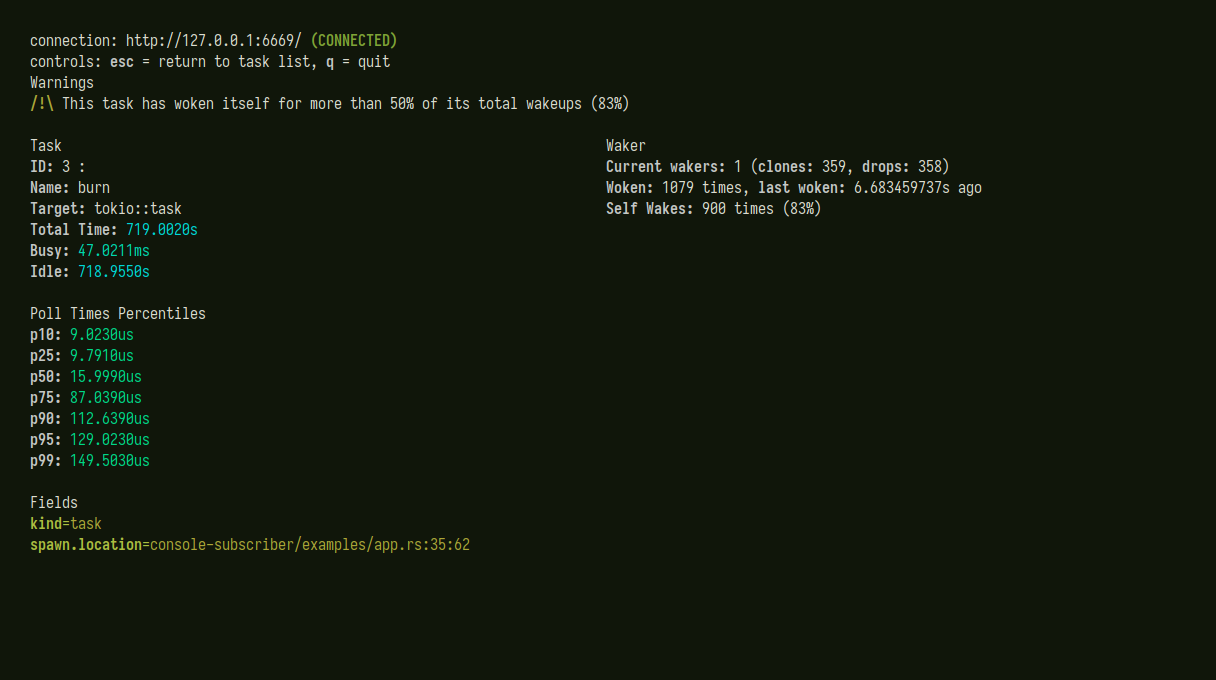 Signed-off-by: Eliza Weisman <eliza@buoyant.io>
- Loading branch information
Showing
7 changed files
with
285 additions
and
90 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.