Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
Contributor Checklist
CHANGELOG.md
dcc.Timer()
The new Timer component is a response to the discussion in #857.
This PR is moved here from dash-core-components PR# 961
dcc.Timer
is based on thedcc.Interval
component. It has all the functionality ofdcc.Interval
plus these new features:countdown
orstopwatch
(count up) modes.'15d 11h 23m 20s' See other available formats in the
timer_format
prop.frequently (ie every interval) just to update a countdown/stopwatch message.
Component Properties
n_intervals
everyinterval
millisecondsduration
and will show the number of milliseconds remaining. When in stopwatch mode, the timer will count up from zero and show the number of milliseconds elapsed. (read only)countdown
mode and count up from zero instopwatch
modefire_times
can reduce the number of times a callback is fired and can increase app performance. The time(s) must be a multiple of the interval.fire_times
list. Usingat_fire_time
in a callback will trigger the callback at the time(s) infire_times
(Read only)duration
.string
message is to be displayed. Note:timer_format
will overridemessages
.messages
. This formats the timer (milliseconds) into human readable formats.messages
will be shown.dcc.Timer demo
🎉 No callbacks required in the first 6 examples! 🎉
Countdown to time of day
Note that the layout is a function so that the timer is updated when the browser is refreshed.
Countdown to date
Countdown timer - repeats every 30 seconds
(partial gif)
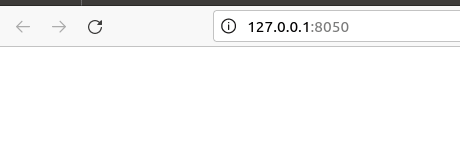
Countdown timer - to target time
Stopwatch - repeats every 30 seconds
(partial gif)
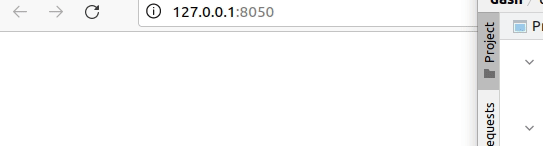
"Stopwatch - with custom messages",
(partial gif- just shows first 30 seconds)
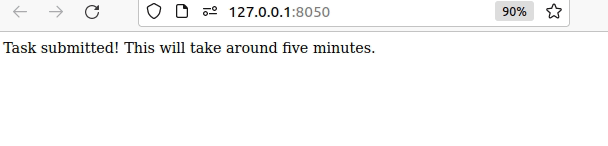
Timing a callback
This example displays how long the callback has been running. When the start button is clicked, one callback starts the timer. A second callback pauses the timer when the callback finishes.
(Note that this only works if you stay on the page while the callback runs)
Timing background callbacks
Space shuttle launch 🚀
This example demos:
at_fire_time
propmessages
prop to display a message at a certain time (without using a callback)dcc.Timer
s in a callback based on user input (in this case, a button to start the countdown sequence)Limitations
When using with multi-page apps, if the timer is on a certain page rather than in
app.py
, the timer will restart when navigating to that page. This could be confusing in cases where the timer is set for a fixed duration. You might expect the timer to keep running when you switch pages (it does not). Timers set for a certain datetime will work as expected on any page of a multi-page app.It's possible to use the
at_fire_time
prop to trigger a callback. However, this is not recommended for scheduling tasks because the user’s tab need to stay open until the specified time in order for the callback to be triggered. In a multi-page app, the callback will not fire unless page with the timer is open.