-
-
Notifications
You must be signed in to change notification settings - Fork 9.4k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feature #27444 [Console] Add Cursor class to control the cursor in th…
…e terminal (pierredup) This PR was squashed before being merged into the 5.1-dev branch. Discussion ---------- [Console] Add Cursor class to control the cursor in the terminal | Q | A | ------------- | --- | Branch? | master | Bug fix? | no | New feature? | yes | BC breaks? | no | Deprecations? | no | Tests pass? | yes | Fixed tickets | N/A | License | MIT | Doc PR | TBD Add a new `Cursor` class to the Console component, that allows to manipulate the cursor in the terminal. Options to move the cursor include: moveUp, moveDown, moveLeft, moveRight, show, hide, save and restore position, get current position etc. Example usage: ```php $cursor = new Cursor($output); $parts = [ ['x' => 9, 'y' => 5, 'string' => '#'], ['x' => 9, 'y' => 7, 'string' => '#'], ['x' => 2, 'y' => 7, 'string' => '#####'], ['x' => 9, 'y' => 1, 'string' => '#######'], ['x' => 7, 'y' => 6, 'string' => '#'], ['x' => 9, 'y' => 2, 'string' => '#'], ['x' => 9, 'y' => 6, 'string' => '#'], ['x' => 9, 'y' => 3, 'string' => '#'], ['x' => 2, 'y' => 1, 'string' => '#####'], ['x' => 7, 'y' => 2, 'string' => '#'], ['x' => 9, 'y' => 4, 'string' => '#####'], ['x' => 1, 'y' => 2, 'string' => '#'], ['x' => 1, 'y' => 6, 'string' => '#'], ['x' => 2, 'y' => 4, 'string' => '#####'], ['x' => 1, 'y' => 3, 'string' => '#'], ['x' => 7, 'y' => 5, 'string' => '#'], ]; foreach ($parts as $part) { $cursor->moveToPosition($part['x'], $part['y']); $output->write($part['string']); usleep(200000); } $cursor->moveToPosition(1, 8); ``` Result: 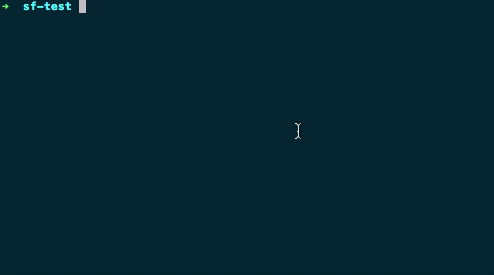 Commits ------- 80d59d5 [Console] Add Cursor class to control the cursor in the terminal
- Loading branch information
Showing
6 changed files
with
361 additions
and
14 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,137 @@ | ||
<?php | ||
|
||
/* | ||
* This file is part of the Symfony package. | ||
* | ||
* (c) Fabien Potencier <fabien@symfony.com> | ||
* | ||
* For the full copyright and license information, please view the LICENSE | ||
* file that was distributed with this source code. | ||
*/ | ||
|
||
namespace Symfony\Component\Console; | ||
|
||
use Symfony\Component\Console\Output\OutputInterface; | ||
|
||
/** | ||
* @author Pierre du Plessis <pdples@gmail.com> | ||
*/ | ||
class Cursor | ||
{ | ||
private $output; | ||
|
||
private $input; | ||
|
||
public function __construct(OutputInterface $output, $input = STDIN) | ||
{ | ||
$this->output = $output; | ||
$this->input = $input; | ||
} | ||
|
||
public function moveUp(int $lines = 1) | ||
{ | ||
$this->output->write(sprintf("\x1b[%dA", $lines)); | ||
} | ||
|
||
public function moveDown(int $lines = 1) | ||
{ | ||
$this->output->write(sprintf("\x1b[%dB", $lines)); | ||
} | ||
|
||
public function moveRight(int $columns = 1) | ||
{ | ||
$this->output->write(sprintf("\x1b[%dC", $columns)); | ||
} | ||
|
||
public function moveLeft(int $columns = 1) | ||
{ | ||
$this->output->write(sprintf("\x1b[%dD", $columns)); | ||
} | ||
|
||
public function moveToColumn(int $column) | ||
{ | ||
$this->output->write(sprintf("\x1b[%dG", $column)); | ||
} | ||
|
||
public function moveToPosition(int $column, int $row) | ||
{ | ||
$this->output->write(sprintf("\x1b[%d;%dH", $row + 1, $column)); | ||
} | ||
|
||
public function savePosition() | ||
{ | ||
$this->output->write("\x1b7"); | ||
} | ||
|
||
public function restorePosition() | ||
{ | ||
$this->output->write("\x1b8"); | ||
} | ||
|
||
public function hide() | ||
{ | ||
$this->output->write("\x1b[?25l"); | ||
} | ||
|
||
public function show() | ||
{ | ||
$this->output->write("\x1b[?25h\x1b[?0c"); | ||
} | ||
|
||
/** | ||
* Clears all the output from the current line. | ||
*/ | ||
public function clearLine(bool $fromCurrentPosition = false) | ||
{ | ||
if (true === $fromCurrentPosition) { | ||
$this->output->write("\x1b[K"); | ||
} else { | ||
$this->output->write("\x1b[2K"); | ||
} | ||
} | ||
|
||
/** | ||
* Clears all the output from the cursors' current position to the end of the screen. | ||
*/ | ||
public function clearOutput() | ||
{ | ||
$this->output->write("\x1b[0J", false); | ||
} | ||
|
||
/** | ||
* Clears the entire screen. | ||
*/ | ||
public function clearScreen() | ||
{ | ||
$this->output->write("\x1b[2J", false); | ||
} | ||
|
||
/** | ||
* Returns the current cursor position as x,y coordinates. | ||
*/ | ||
public function getCurrentPosition(): array | ||
{ | ||
static $isTtySupported; | ||
|
||
if (null === $isTtySupported && \function_exists('proc_open')) { | ||
$isTtySupported = (bool) @proc_open('echo 1 >/dev/null', [['file', '/dev/tty', 'r'], ['file', '/dev/tty', 'w'], ['file', '/dev/tty', 'w']], $pipes); | ||
} | ||
|
||
if (!$isTtySupported) { | ||
return [1, 1]; | ||
} | ||
|
||
$sttyMode = shell_exec('stty -g'); | ||
shell_exec('stty -icanon -echo'); | ||
|
||
@fwrite($this->input, "\033[6n"); | ||
|
||
$code = trim(fread($this->input, 1024)); | ||
|
||
shell_exec(sprintf('stty %s', $sttyMode)); | ||
|
||
sscanf($code, "\033[%d;%dR", $row, $col); | ||
|
||
return [$col, $row]; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.