Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Browse files
Browse the repository at this point in the history
# Reverts #43587 PR #43587 breaks the `placeholder="blur"` property on the `<Image />` component by keeping the `blurStyles`, e.g. the blurred image, after the image is loaded. **This regression does _not_ introduce any breaking changes or bugs.** --- The reason for the original PR was: > This PR remove `React.useState()` from the `next/image` component. It was only used in the `onError` case and it was causing Safari to become very slow when there were many images on the same page. We were seeing 1s delay blocking the main thread when there were about 350 images on the same page. Chrome and Firefox were not slow. The original PR is a performance improvement for Safari on a corner case. Additionally, when tackling this performance improvement again, the `blurStyle` needs to know when the the image is done loading so it can get rid of the blur. The state is updated in `handeLoading()` and isn't just used `onError`. ## Fixes issues - Fixes #43829 - Fixes #43689 ## To reproduce For reference this when #43587 was pulled into Next.js [v13.0.6-canary.3](https://github.com/vercel/next.js/blob/v13.0.6-canary.3/packages/next/client/image.tsx) - Regress the `image.tsx` to [v13.0.6-canary.2](https://github.com/vercel/next.js/blob/v13.0.6-canary.2/packages/next/client/image.tsx) - Do a local build with the regressed `image.tsx` on (current canary build) [v13.0.8-canary.0](https://github.com/vercel/next.js/releases/tag/v13.0.8-canary.0) - Example code, (import any image you like) make sure to use `placeholder="blur"` ```typescript import Image from 'next/image' import CatImage from '../public/cat.png' <Image src={CatImage} width={500} height={500} alt="Cat" priority placeholder="blur" /> ``` - Image will still have the blur after the image is loaded - Before and after screenshot 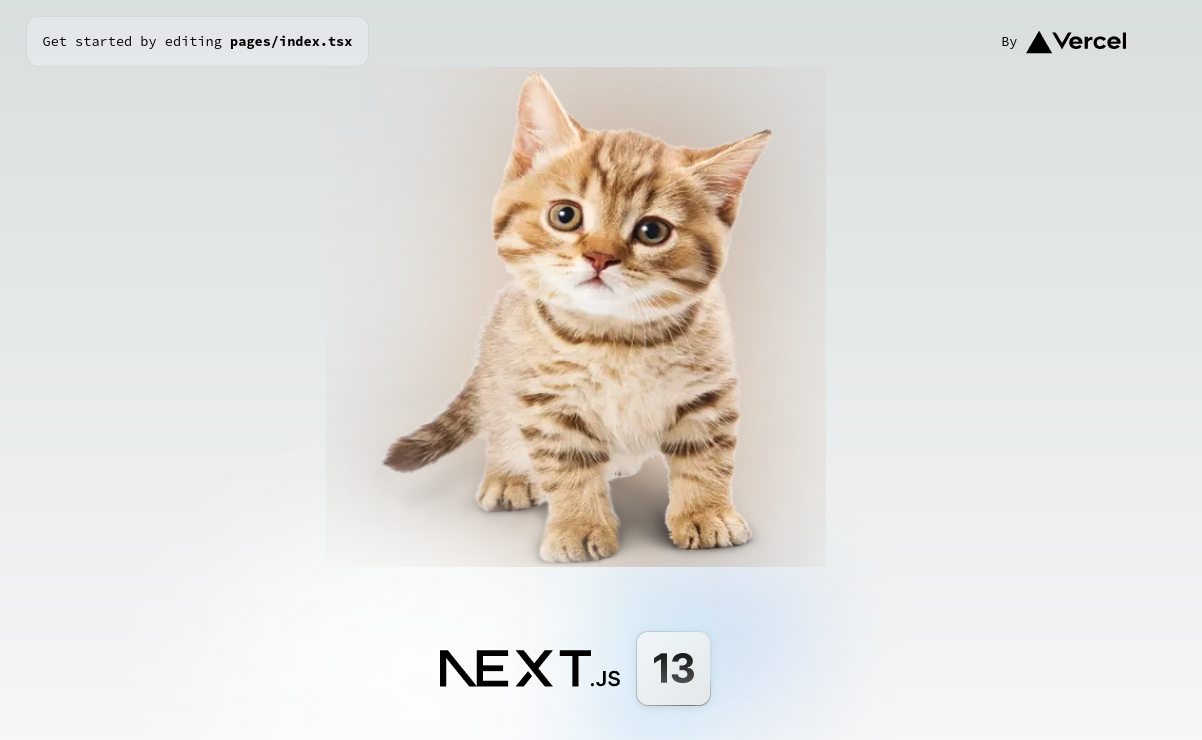 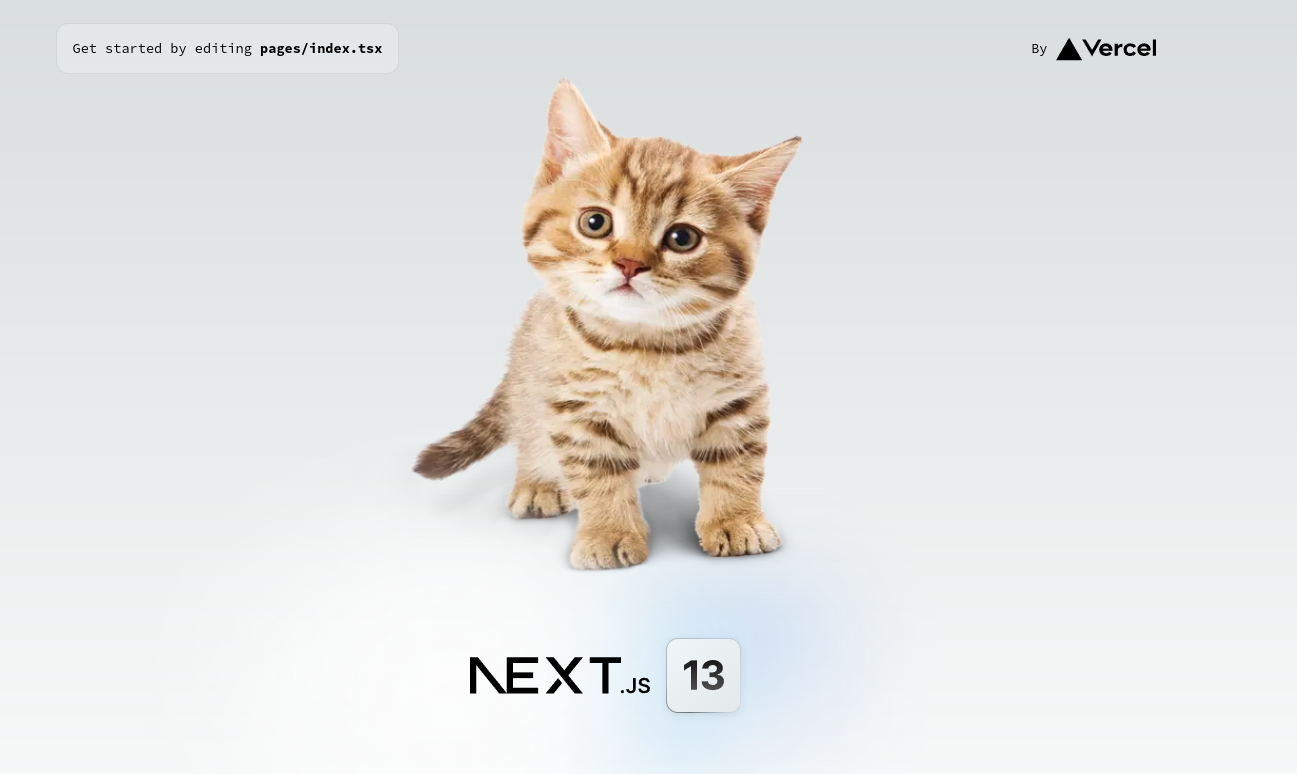 Co-authored-by: Steven <steven@ceriously.com>
- Loading branch information
Showing
4 changed files
with
141 additions
and
43 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
31 changes: 31 additions & 0 deletions
31
test/integration/next-image-new/default/pages/blurry-placeholder.js
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,31 @@ | ||
import React from 'react' | ||
import Image from 'next/image' | ||
|
||
export default function Page() { | ||
return ( | ||
<div> | ||
<p>Blurry Placeholder</p> | ||
|
||
<Image | ||
priority | ||
id="blurry-placeholder-raw" | ||
src="/test.ico" | ||
width="400" | ||
height="400" | ||
placeholder="blur" | ||
blurDataURL="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAYAAAAfFcSJAAAADUlEQVR42mP8P4nhDwAGuAKPn6cicwAAAABJRU5ErkJggg==" | ||
/> | ||
|
||
<div id="spacer" style={{ height: '1000vh' }} /> | ||
|
||
<Image | ||
id="blurry-placeholder-with-lazy" | ||
src="/test.bmp" | ||
width="400" | ||
height="400" | ||
placeholder="blur" | ||
blurDataURL="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAYAAAAfFcSJAAAADUlEQVR42mO0/8/wBwAE/wI85bEJ6gAAAABJRU5ErkJggg==" | ||
/> | ||
</div> | ||
) | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters